Fantasy FRC
Project Overview
Fantasy FRC is fantasy football with robotics. It is a website that manages the full process of running a draft for FRC, and it's pretty customizable.
If you would like to check it out, the full code is available on GitHub: Fantasy FRC.
Modifying
The source code is protected under the MIT license, so you have full rights to modify it as long as you keep the licenses and copyright intact. NOTE: I am not an expert in JS, and while I have done my best to weed out any bugs, there might still be some out there. If you find any, please open an issue on GitHub or create a pull request with a fix.
Installation
Download Source Code and Required Software
Download the source code from the GitHub repository and put it on whatever computer you want to run the website. I used a dedicated machine running Ubuntu Server, but the website is not very intense, so any machine should work.
Install Node.js (Windows | Ubuntu). If on Linux, navigate to /server, uninstall bcrypt, and reinstall it using npm. This is needed because the Windows and Linux versions are different.
Install MySQL (Windows | Ubuntu) and go through initial setup.
Setup Database and APIs
Login to your MySQL server and create a database, which I called fantasy
. And create two tables for users and teams.
Create users table:
CREATE TABLE users (
name VARCHAR(255),
passw CHAR,
id VARCHAR(255),
position INT,
score DOUBLE,
teams TEXT
);
Create teams table:
CREATE TABLE teams (
name VARCHAR(255),
number INT,
average DOUBLE,
opr DOUBLE,
score DOUBLE,
location VARCHAR(255),
owner VARCHAR(255)
);
Get a Blue Alliance API key from their website.
Create server_info.ini in /server and add the required fields to it, it should look like this:
[SQL]
SQL_Passw = "password" # Password for MySQL server
SQL_IP = "192.168.1.1" # Hostname for MySQL server
SQL_User = "username" # Username for MySQL serer
SQL_Database = "fantasy" # Database name for MySQL server
[TBA]
BLUE_ALLIANCE = supercoolapikey # API key for Blue Alliance website (do not put it in quotes)
[SERVER]
SECRET = "super secret" # secret for sessions (I made mine a random string)
Setup Website
Navigate to /server/server.js, and locate app.get('/admin', (req, res) => {
. This is the code for serving the admin page. As there are no admin users we will need to disable security for the admin page to add them.
Change:
app.get('/admin', (req, res) => {
try {
if (req.isAuthenticated()) {
user = Object.values(JSON.parse(JSON.stringify(req.user[0])))[1];
if (user === adminName) {
res.sendFile('www/admin.html', { root: __dirname });
}
else {
res.sendStatus(403);
}
}
else {
res.redirect('/');
}
}
catch (error) {
console.log("ERROR:");
console.log(error);
res.sendStatus(500);
}
});
To this:
app.get('/admin', (req, res) => {
try {
// if (req.isAuthenticated()) {
// user = Object.values(JSON.parse(JSON.stringify(req.user[0])))[1];
// if (user === adminName) {
res.sendFile('www/admin.html', { root: __dirname });
// }
// else {
// res.sendStatus(403);
// }
// }
// else {
// res.redirect('/');
// }
}
catch (error) {
console.log("ERROR:");
console.log(error);
res.sendStatus(500);
}
});
* If you want to connect to the website externally you will need to port forward port 80 to your web server.
Run the website using node server.js
(server.js in /server) and navigate to <hostname>/admin
(the admin page is only accessible by direct link) and type in a username and password. Once your admin has been added uncomment the lines to re-enable security. Then go to your MySQL server and get the ID and name of the account. In server.js locate const adminId =
and set adminId
and adminName
to the user's ID and name respectively. Once an admin user is set up your admin go to the admin page and add the rest of your users.
Yearly Setup
The setup for the 2023 season is already done but if you need to redo it for any reason here is the process.
- Update the year in both rolling_team_info.py and get_team_info.py
- Run /server/get_team_info.py and move the generated team_info.json to /server
Run
To run the server you can use node server.js
(server.js is in /server) to run the server and view the output. If you do not need to see the output and you are running the website on a dedicated machine you can use forever.
How does the draft work
The draft starts when the Start Draft
button is pressed on the admin page. As soon as it is pressed all users will get access to the drafting page and a 5-minute countdown is started. Once the countdown ends the draft will start and users will get a chance to pick teams. Each user gets 20 seconds to pick a team and each team can only be picked once. Users can only have one team from each location (location is country or state if it is a USA team). If a user picks a team with a duplicate location the team will get removed from their queue and the 20-second timer will get reset (NOTE a new team will not be chosen until the user adds another team). For Example:
- A user picks a team with a location they already have drafted
- The server rejects their choice and the timer is reset and the rejected team is removed
- The user removes the team at the top of their queue because they are not ready to pick them
- The user presses the
Add
button on any team and the team at the top of their queue is chosen
How do teams get a score
Run /server/rolling_team_info.py
and it will update all team's and user's scores. It will need to be run regularly to keep things up to date.
Showcase
Home
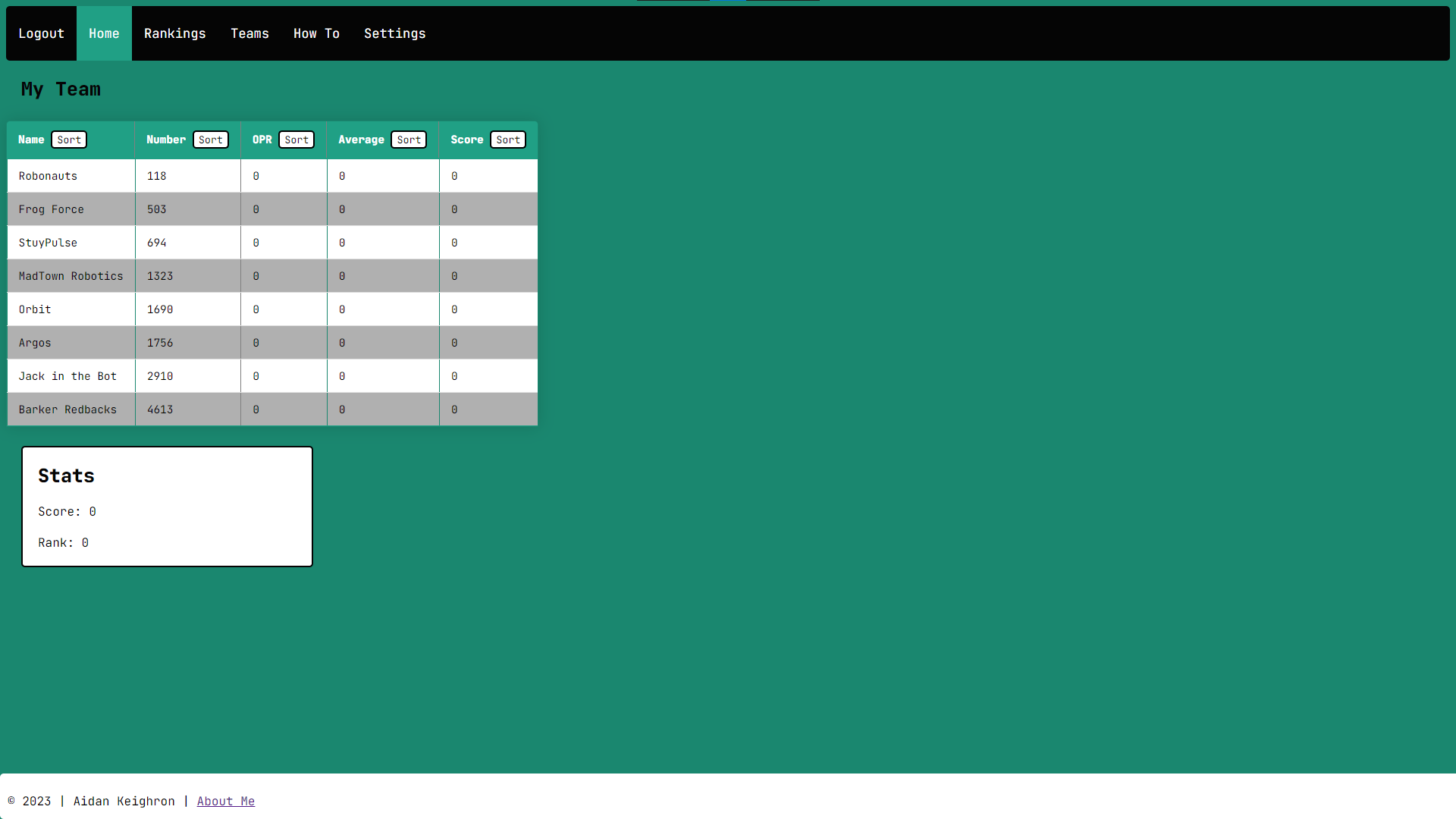
Teams
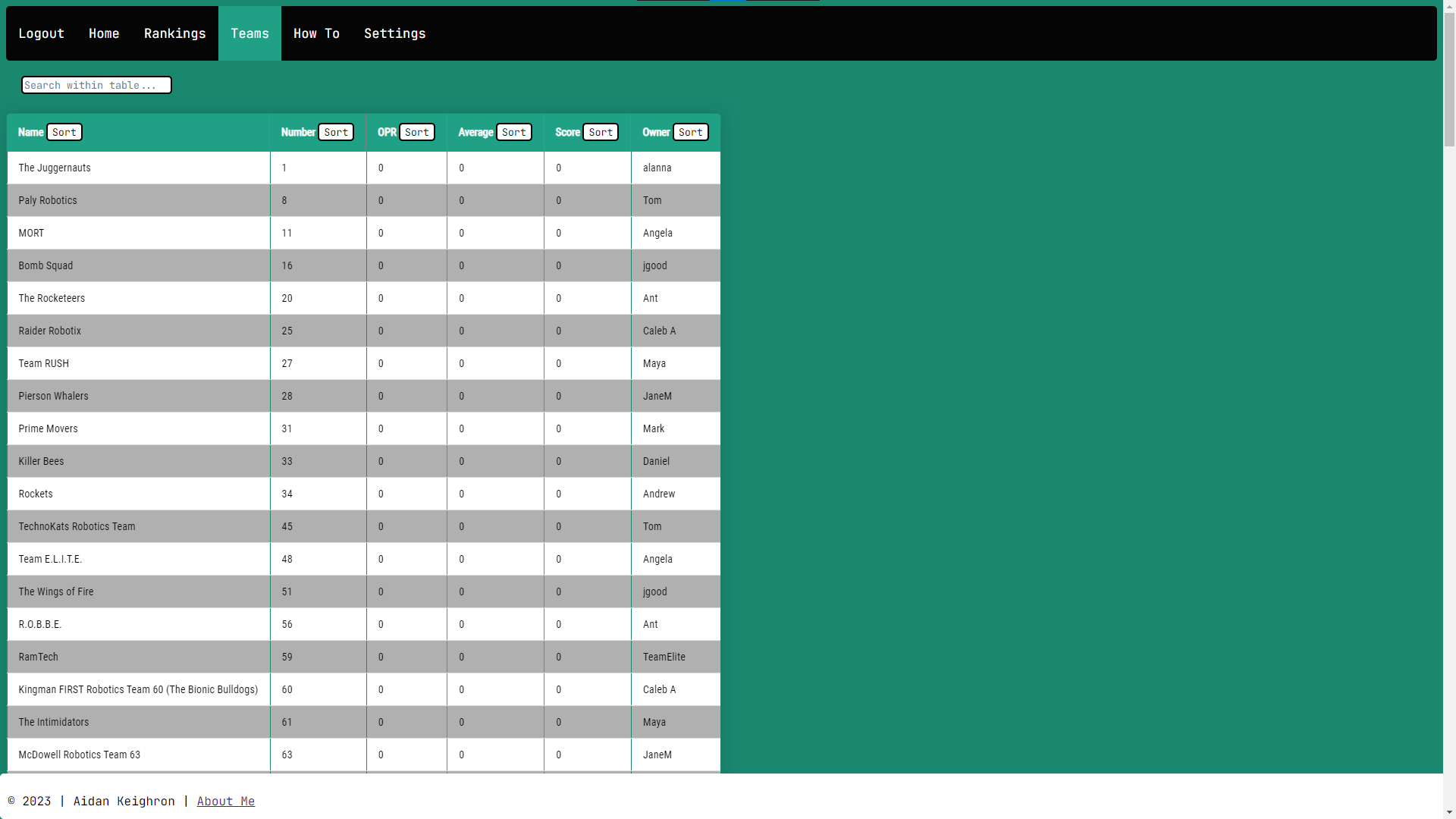
Rankings
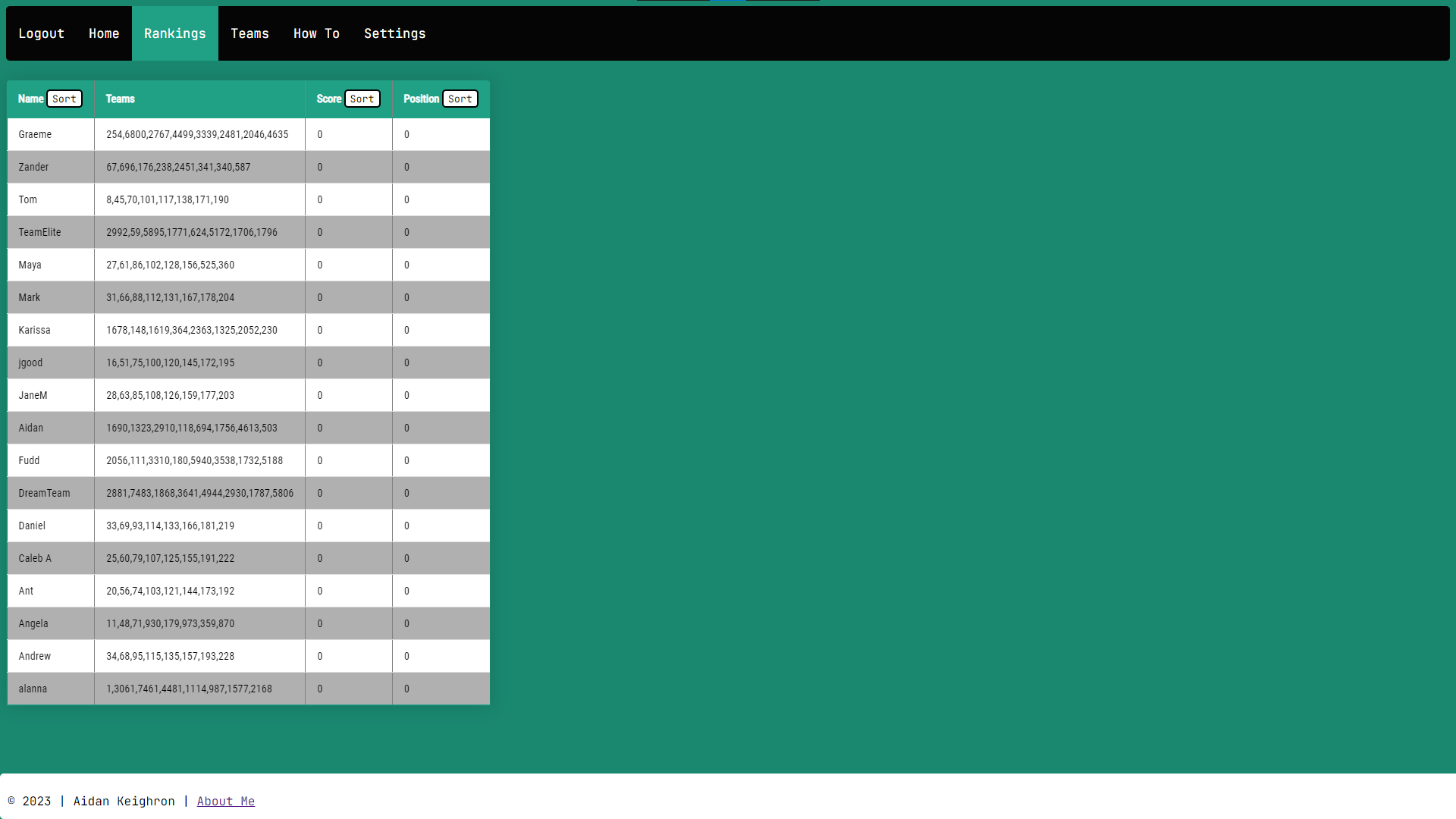
Login
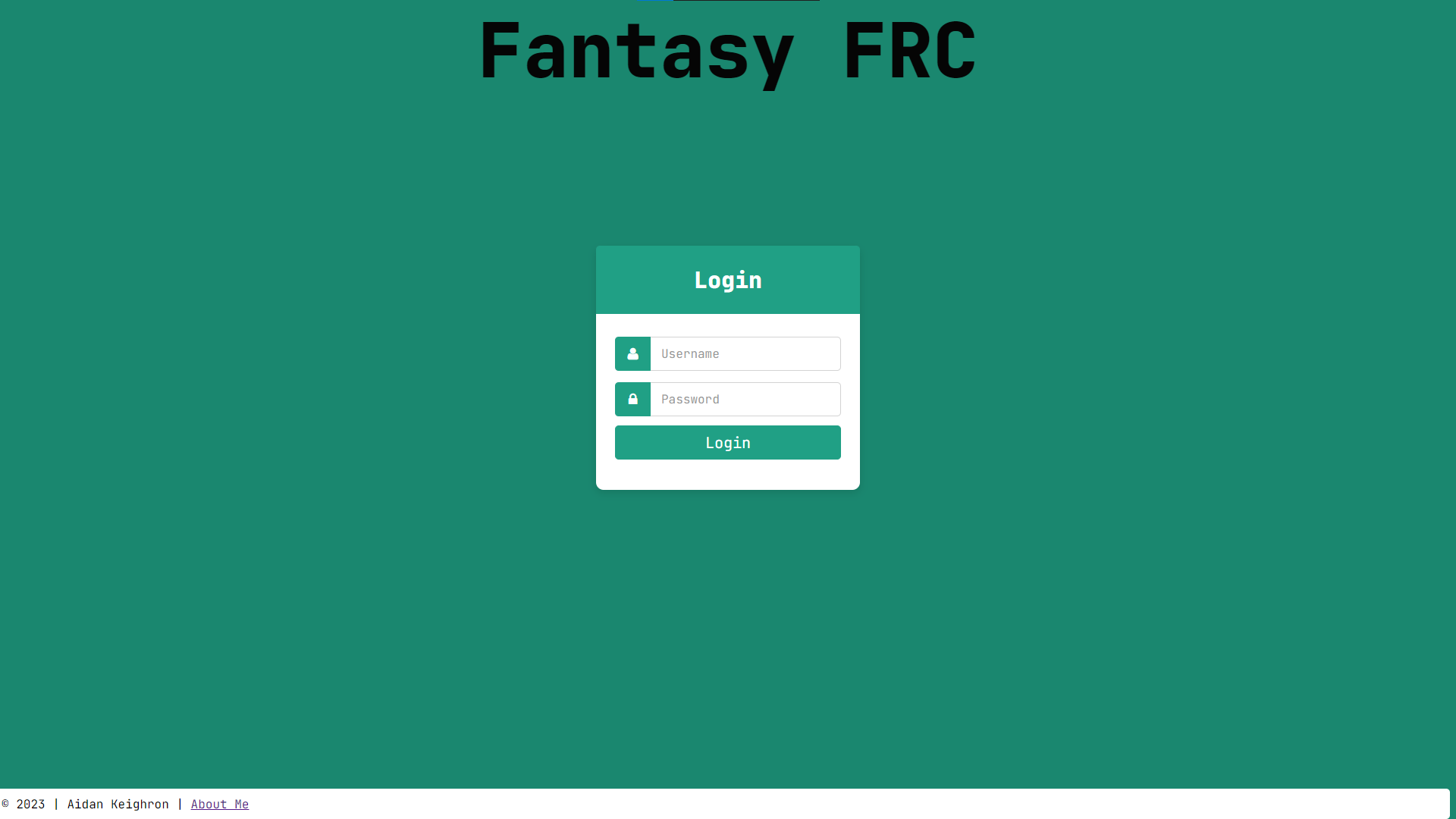
Settings
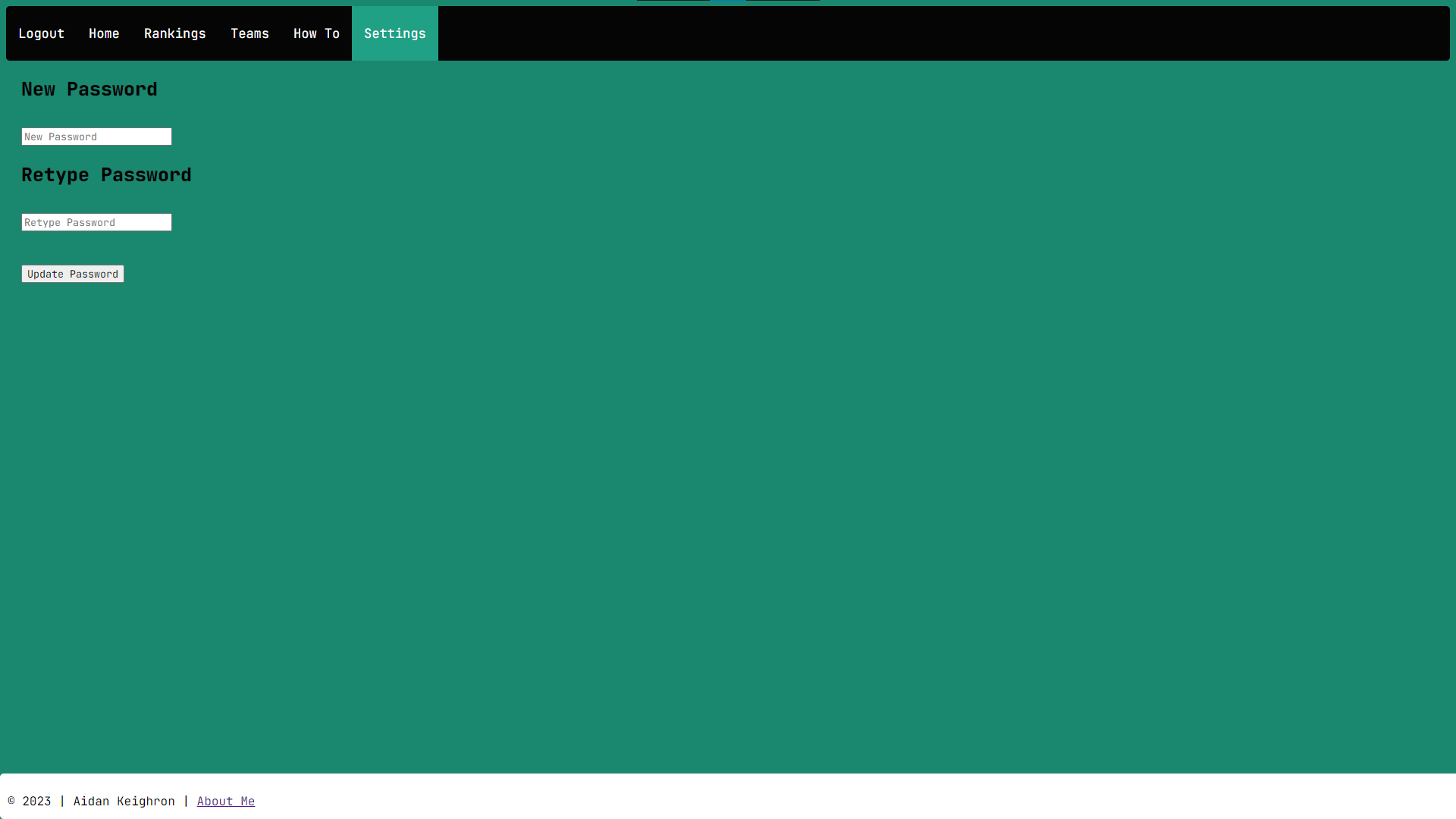
How To
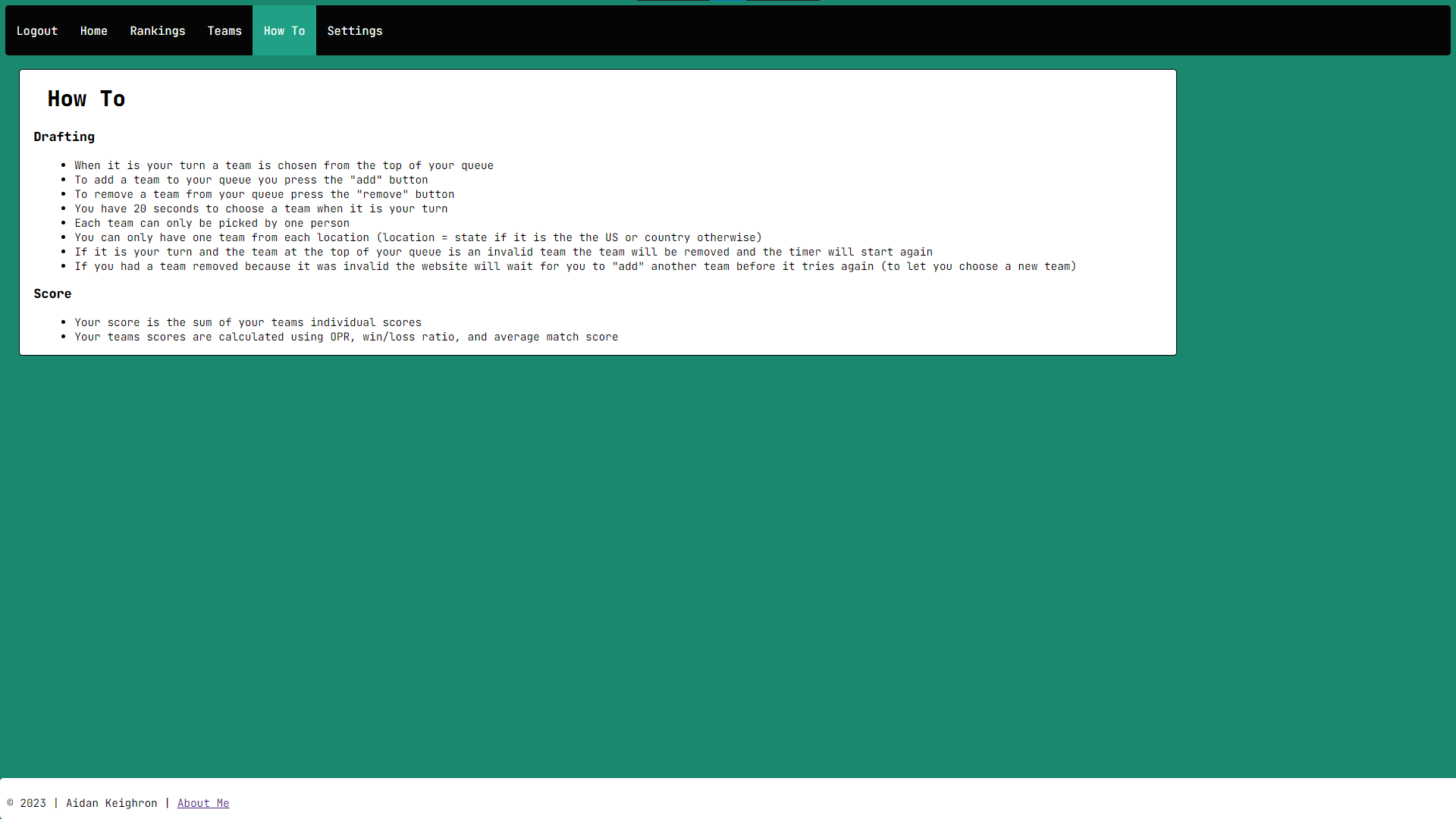
Admin
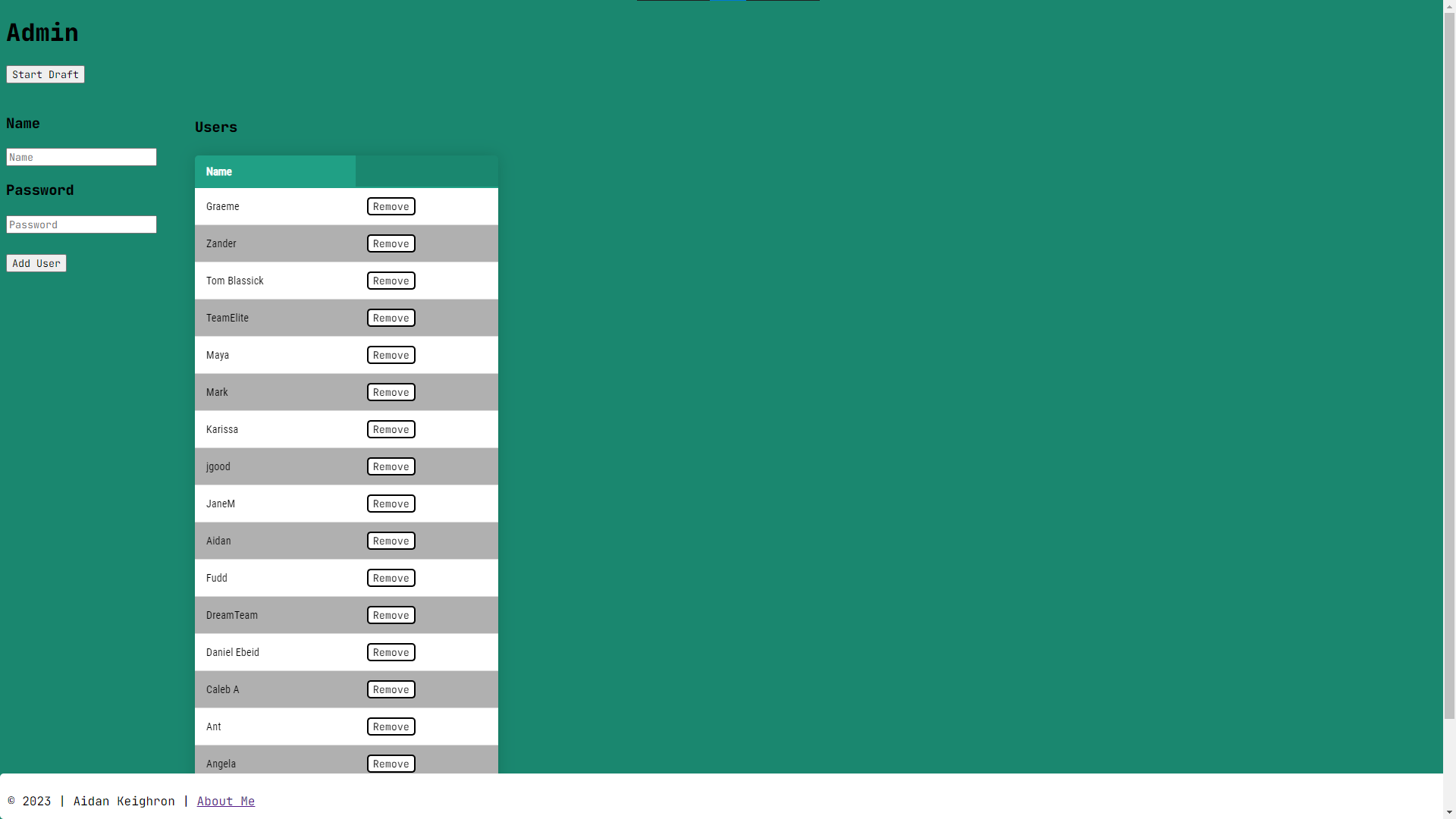
Drafting
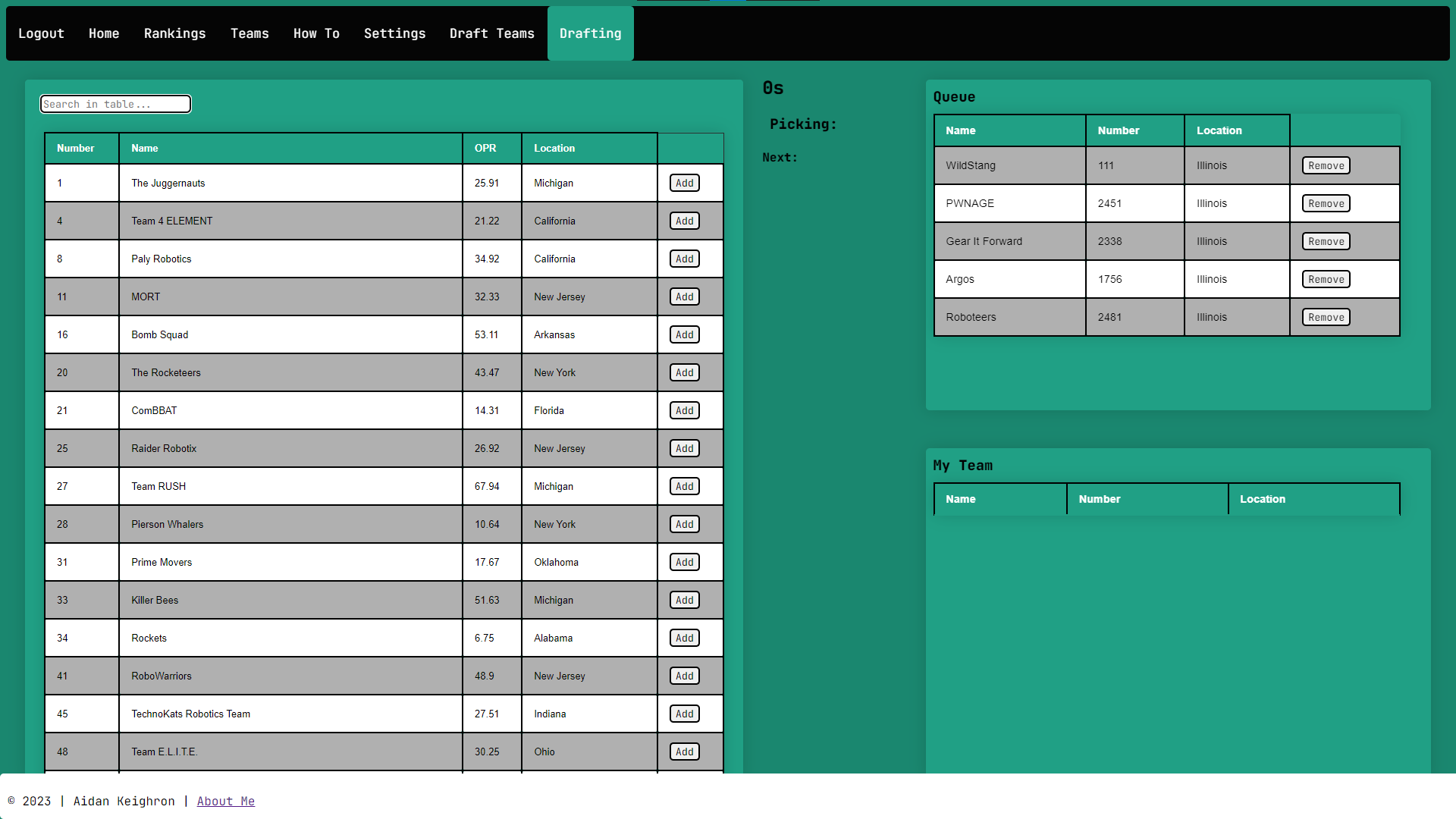
Search
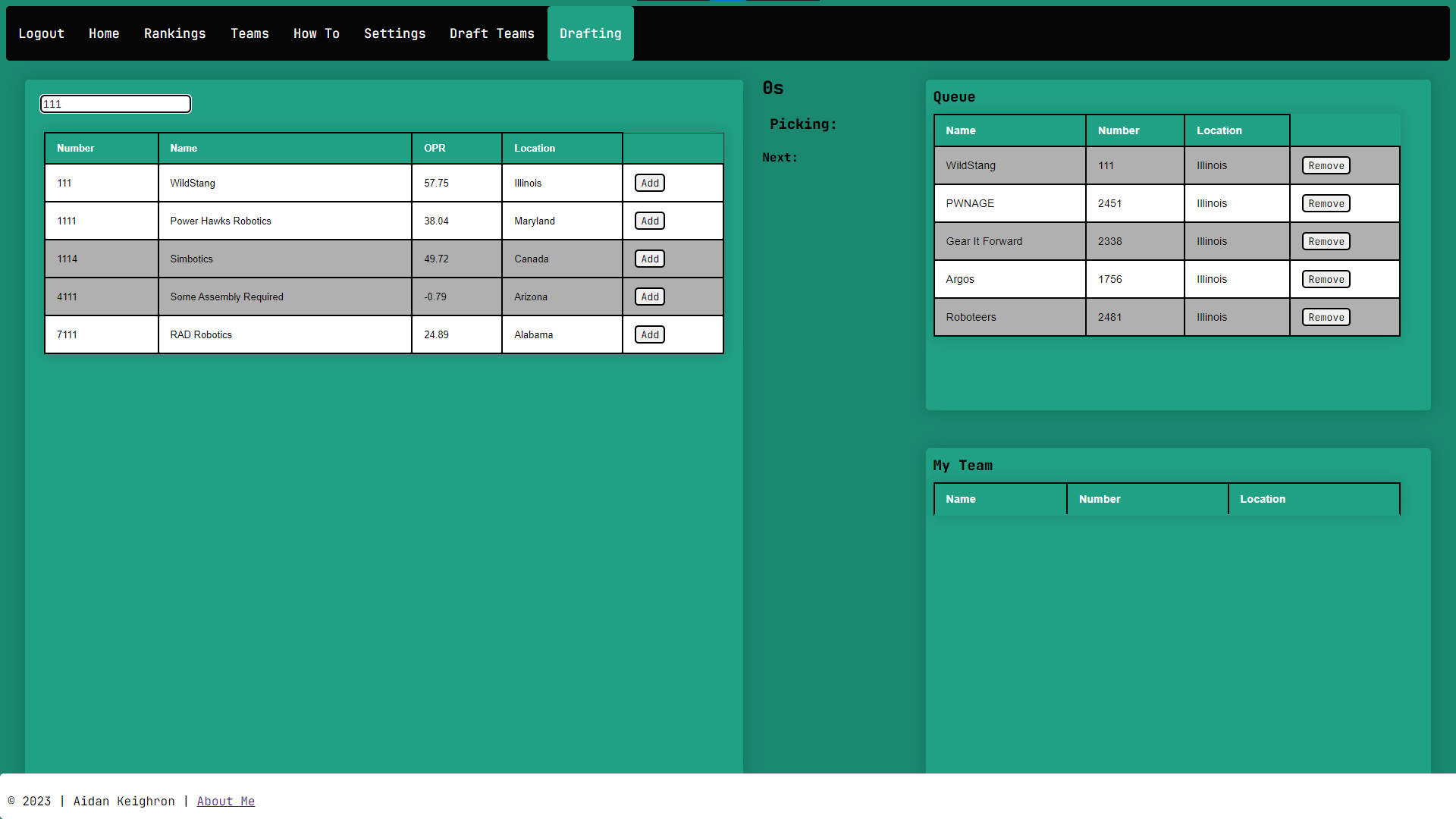
On the drafting page and teams page there is a search option that lets you search using team numbers or names.
If you would like to check it out, the full code is available on GitHub: Fantasy FRC.